An Observation About Exam. Question 9
A Run
This code compiles and runs, the tree diagram drawing would even look appropriate. However, as pointed out to me, nodes 6 and 7 are not properly send-receive paired.
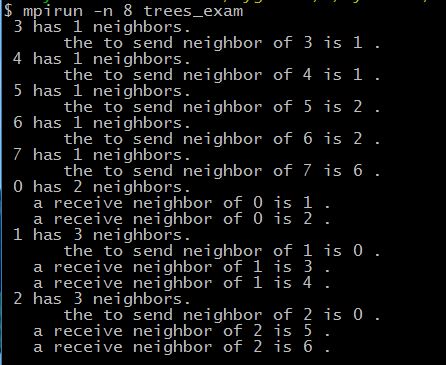
/*
int MPI_Graph_create(MPI_Comm oldcomm, int nnodes, int *index, int *edges, int reorder, MPI_Comm *graphcomm),
where:
oldcomm - the initial communicator,
nnodes - the number of the graph vertices,
index - the number of the arcs proceeding from each vertex,
edges - the sequential list of the graph arcs,
reorder - the flag for pointing out if the process ranks can be reodered,
cartcomm - the created communicator with the graph type topology.
*/
#include<mpi.h>
#include<stdio.h>
/* An 8 node binary tree*/
int main(int argc, char *argv[]) {
MPI_Status status;
int rank,i,j,tag_starter=34;
MPI_Init(&argc, &argv);
MPI_Comm_rank(MPI_COMM_WORLD,&rank);
int reorder=1; /* Can the processes be reordered, may result in improved performance.*/
/*The correct Send-Receive Data
int index[] = {2,5,8,9,10,11,13,14};
int edges[] = {1,2,0,3,4,0,5,6,1,1,2,2,7,6};
*/
int index[] = {2,5,8,9,10,11,12,13};
int edges[] = {1,2,0,3,4,0,5,6,1,1,2,2,6};
int nneighbors, send_rank=-1,receive_ranks[]={-1,-1};
int tags[8][8];
for (i=0;i<8;i++) {
for (j=0;j<8;j++) {
(i<j)?tags[i][j]=tag_starter++:0;
}
}
int * intptr=&nneighbors;
int the_neighbors[10];
MPI_Comm MPI_Tree_World;
MPI_Graph_create(MPI_COMM_WORLD, 8, index, edges, reorder,&MPI_Tree_World);
/*int MPI_Graph_neighbors_count(MPI_Comm comm,int rank, int *nneighbors); */
MPI_Graph_neighbors_count(MPI_Tree_World,rank, intptr);
/*int MPI_Graph_neighbors (MPI_Comm comm,int rank,int mneighbors, int *neighbors); */
MPI_Graph_neighbors (MPI_Tree_World,rank,nneighbors, the_neighbors);
printf(" %i has %i neighbors.\n",rank,nneighbors);
j=0;
for (i=0;i<nneighbors;i++) {
if (the_neighbors[i]<rank) {
send_rank=the_neighbors[i];
} else {
receive_ranks[j++]=the_neighbors[i];
}
}
if (send_rank!=-1) printf(" the to send neighbor of %i is %i .\n",rank,send_rank);
for (i=0;i<2;i++) {
if (receive_ranks[i]!=-1) printf (" a receive neighbor of %i is %i .\n",rank, receive_ranks[i]);
}
MPI_Finalize();
}